Google Pay API
Note
Google Pay is currently a closed Beta feature and does not support CAD at this time.
We support the use of Google Pay on your platform through an API integration. Using Google Pay allows your Merchants to simplify the checkout flow, making checkouts faster, and impacting the conversion performance of your platform.
Payments are encrypted by Google and decrypted by us, protecting payment information even in the event of a compromised application. Follow this guide to learn how to integrate Google Pay in to your platform.
See the image below for a graphic of the entire integration flow.
Note
Setup your Google Merchant Account
Onboard your account to Google
Sign in using your business email address and register your business for a Google account on the Google Pay Business console page, where you will start off by providing your business name, location of operation, and agree to Google's Terms of Service.Further details are outlined in Google's Integration checklist.
Once you've registered your account, navigate to the Business Profile within the console. This is where you will finish Google onboarding, which is a necessary step in submitting your application for approval. Additional application approval steps are outlined in the Submit your App for Production section below.
Access your Google Merchant ID
Once your website passes the initial Google on-boarding review, grab your Google Pay merchant identifier which can be found by clickingDashboard
in the left-hand navigation, located in the top right-hand corner.You will use your Google Merchant ID
later on in your integration.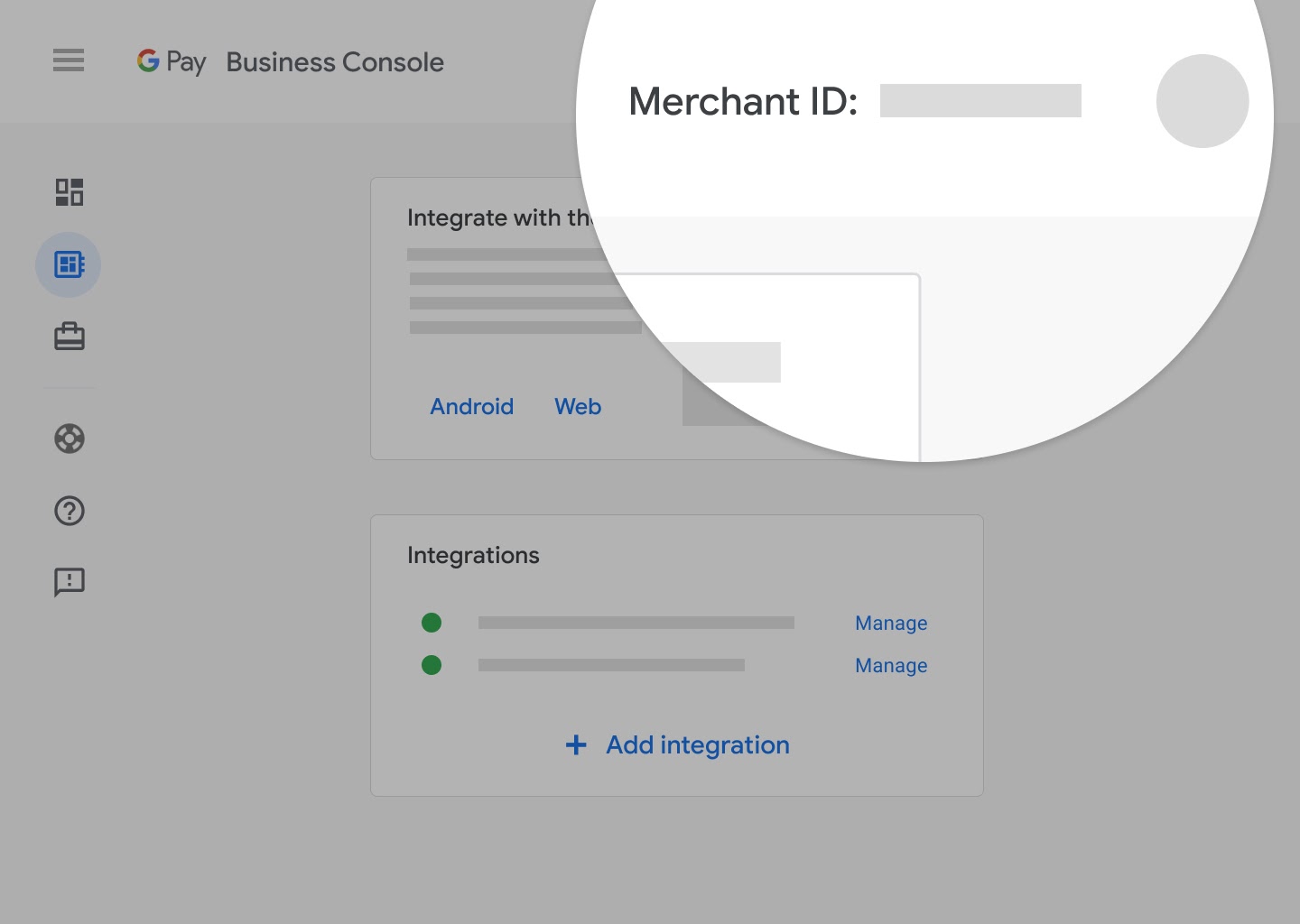
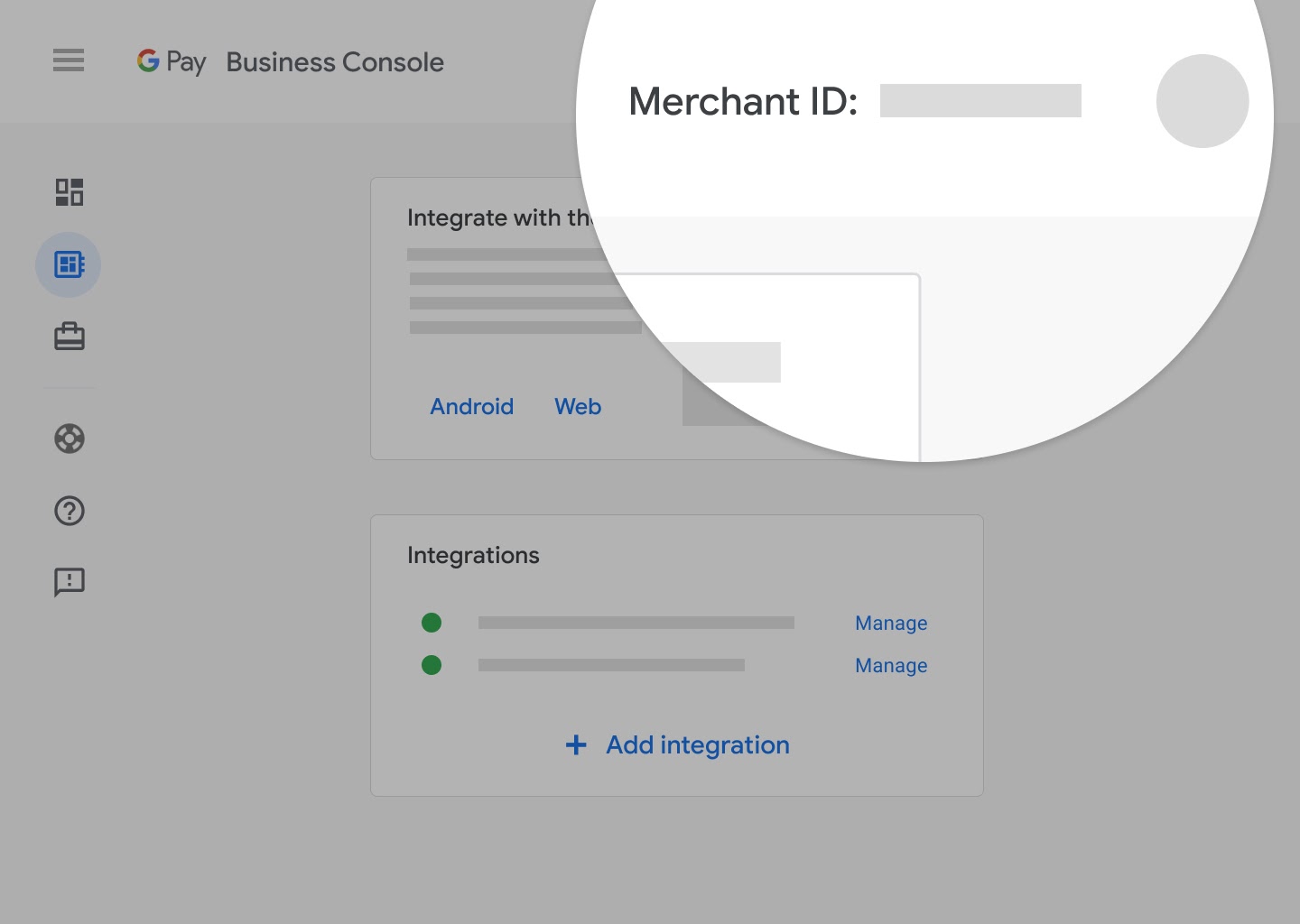
Integrate the Google APIs
Follow Google's integration guide for step-by-step instruction on how to implement Google Pay within your application. The instructions below highlight key configuration settings you will need to include while integrating Google Pay. Alternatively, watch Google's tutorial video to integrate: All about integrating Google Pay online.Configuration Information
Define the version of the Google Pay API when creating your configuration function.
const baseRequest = {
apiVersion: 2,
apiVersionMinor: 0
};
allowedCardNetworks
variable within your application.The Google Pay API might return cards on file with Google (
PAN_ONLY
), and/or a device token on an Android device that's authenticated with a 3-D Secure cryptogram (CRYPTOGRAM_3DS). Instantiate this through the allowedCardAuthMethods
parameter.const allowedCardNetworks = ["AMEX", "DISCOVER", "INTERAC", "JCB", "MASTERCARD", "VISA"];
const allowedCardAuthMethods = ["PAN_ONLY", "CRYPTOGRAM_3DS"];
wepay
within your tokenization specification variable by setting the gateway name to wepay
and the gatewayMerchantId
to your gateway merchant ID. You will receive your gatewayMerchantId
from your WePay integration team.const tokenizationSpecification = {
type: 'PAYMENT_GATEWAY',
parameters: {
'gateway': 'wepay',
'gatewayMerchantId': 'WePay_Merchant_Id'
}
};
Create a Wepay Payment Method
Once your application is able to create Google Pay tokens, you will need to send aPOST /payment_methods
request to us, using the Google Pay token returned to your server. See the sample request below, where the Google Pay token, payment method
type
, and cardholder information need to be provided. Insert the public_key
value received from us during your application on-boarding. Use the https://stage-api.wepay.com
url for staging and the https://api.wepay.com
url for production. See our Process Payments article for further information on processing payments.
Note
3.0.rc.2.1
or 3.2
in the Version header parameter for your requests to this endpoint.POST /payment_methods
curl -X POST \
--url 'https://stage-api.wepay.com/payment_methods' \
-H 'Accept: application/json'\
-H 'App-Id: {YOUR-APP-ID}'\
-H 'App-Token: {YOUR-APP-TOKEN}'\
-H 'Api-Version: '3.2'\
-H 'Content-Type: application/json'\
-H 'Unique-Key: {UNIQUE-KEY}
--data-raw {
"type": "google_pay",
"google_pay": {
"payment_method_data": "BASE_64_ENCODED_RESPONSE_FROM_GOOGLE",
"card_holder": {
"holder_name": "John Snow",
"email": "example@wepay.com",
"address": {
"country": "US",
"postal_code": "94025"
}
}
}
}
Below is a sample response payload where a WePay payment method ID will be returned (be sure to keep this handy - you'll need to use this to create and capture a payment later), as well as fill in other information.
POST /payment_method
{
"id": "00000000-6363-0000-0000-00521ec2df84",
"resource": "payment_methods",
"path": "/payment_methods/00000000-6363-0000-0000-00521ec2df84",
"owner": {
"id": "27415",
"resource": "applications",
"path": null
},
"create_time": 1629930873,
"type": "google_pay",
"google_pay": {
"card_holder": {
"holder_name": "Tony Stark",
"email": "example@wepay.com",
"address": {
"line1": null,
"line2": null,
"city": null,
"region": null,
"postal_code": "94025",
"country": "US"
},
"phone": {
"country_code": null,
"phone_number": null,
"type": null
}
},
"expiration_month": 4,
"expiration_year": 2030,
"display_name": "MasterCard xxxxxx4769"
},
"custom_data": null,
"api_version": "3.0"
}
Create a Wepay Payment
Use the WePay payment method ID returned above in thePOST /payment_methods
type
parameter to "payment_method_id"
when executing Google payments.See our Capture Authorized Payments guide to learn about how to execute manual, deferred, and partial captures of payments.curl -X POST \
--url 'https://stage-api.wepay.com/payments' \
-H 'Accept: application/json'\
-H 'App-Id: {YOUT-APP-ID}'\
-H 'App-Token: {YOUR-APP-TOKEN}'\
-H 'Api-Version: 3.0'\
-H 'Content-Type: application/json'\
-H 'Unique-Key: {UNIQUE-KEY}
--data-raw '{
"amount": AMOUNT_AS_INTEGER,
"currency": "USD",
"account_id": "{merchant's-account-id}",
"payment_method": {
"payment_method_id": "INSERT_PAYMENT_METHODS-ID",
"type": "payment_method_id"
}
}
Submit your App for Production
There are two final steps before your platform is fully approved for production.
Certify with Google
Before your platform can fully integrate with Google, the Google Pay team must clear your final integration for production. After you have completed each part of the integration checklist, which includes testing your integration, navigate to your Google Pay Business console to get started.- Click "Integrations" in the left-hand navigation.
- Click "Integrate with your website."
- Click "Add a new website" and insert the production website URL of your Platform.
- For "Integration type" choose "Gateway."
- Upload required screenshots to show what the integration looks like.
After your platform has been approved for Production by Google, you will receive a confirmation email.
Certify with WePay
To ensure that we can decrypt your Google Pay Token, test the flow from creating the Google Pay Token to using that token to create a WePay Payment Method. No manual intervention from us is required for this certification, as successfully creating the WePay Payment Method inherently validates that we are able to decrypt the token.